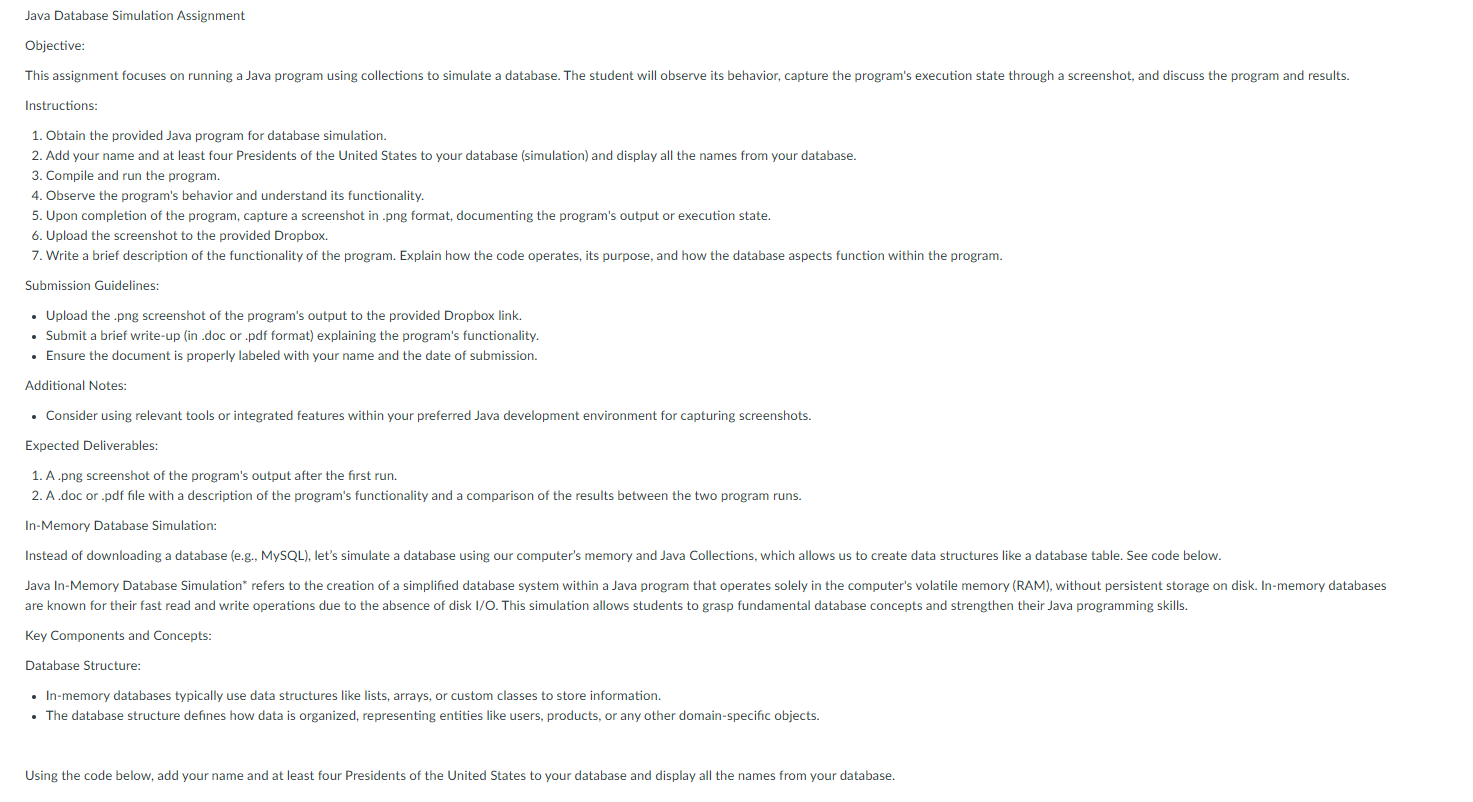
import java.util.HashMap;
import java.util.Map;
public class InMemoryDatabaseSimulation {
private Map<String, Map<Integer, String>> tables;
public InMemoryDatabaseSimulation() {
tables = new HashMap<>();
}
public void createTable(String tableName) {
tables.put(tableName, new HashMap<>());
}
public void insertData(String tableName, int id, String data) {
if (tables.containsKey(tableName)) {
tables.get(tableName).put(id, data);
} else {
System.out.println("Table '" + tableName + "' does not exist.");
}
}
public String fetchData(String tableName, int id) {
if (tables.containsKey(tableName)) {
return tables.get(tableName).get(id);
} else {
System.out.println("Table '" + tableName + "' does not exist.");
return null;
}
}
public static void main(String[] args) {
InMemoryDatabaseSimulation database = new InMemoryDatabaseSimulation();
// Create a table
database.createTable("users");
// Insert data
database.insertData("users", 1, "John Doe");
database.insertData("users", 2, "Jane Doe");
// Fetch data
System.out.println("User 1: " + database.fetchData("users", 1));
System.out.println("User 2: " + database.fetchData("users", 2));
System.out.println("User 3: " + database.fetchData("users", 3)); // Non-existent entry
}
}
Objective: Instructions: 1. Obtain the provided Java program for database simulation. 2. Add your name and at least four Presidents of the United States to your database (simulation) and display all the names from your database. 3. Compile and run the program. 4. Observe the program's behavior and understand its functionality. 5. Upon completion of the program, capture a screenshot in .png format, documenting the program's output or execution state. 6. Upload the screenshot to the provided Dropbox. 7. Write a brief description of the functionality of the program. Explain how the code operates, its purpose, and how the database aspects function within the program. Submission Guidelines: - Upload the .png screenshot of the program's output to the provided Dropbox link. - Submit a brief write-up (in .doc or .pdf format) explaining the program's functionality. - Ensure the document is properly labeled with your name and the date of submission. Additional Notes: - Consider using relevant tools or integrated features within your preferred Java development environment for capturing screenshots. Expected Deliverables: 1. A.png screenshot of the program's output after the first run. 2. A.doc or .pdf file with a description of the program's functionality and a comparison of the results between the two program runs. In-Memory Database Simulation: are known for their fast read and write operations due to the absence of disk I/O. This simulation allows students to grasp fundamental database concepts and strengthen their Java programming skills. Key Components and Concepts: Database Structure: - In-memory databases typically use data structures like lists, arrays, or custom classes to store information. - The database structure defines how data is organized, representing entities like users, products, or any other domain-specific objects. Using the code below, add your name and at least four Presidents of the United States to your database and display all the names from your database.