You have been tasked to write a C++ program for a simple text-based 2-person role-playing game (RPG). Read all these instructions carefully and completely before starting to write any code.
Input File and Game Setup
The character data and game parameters will be specified in an input text file named rpg-data.txt. The input file will contain 6 lines with the following format. Note that in the template below "P1" refers to Player 1 and "P2" refers to Player 2
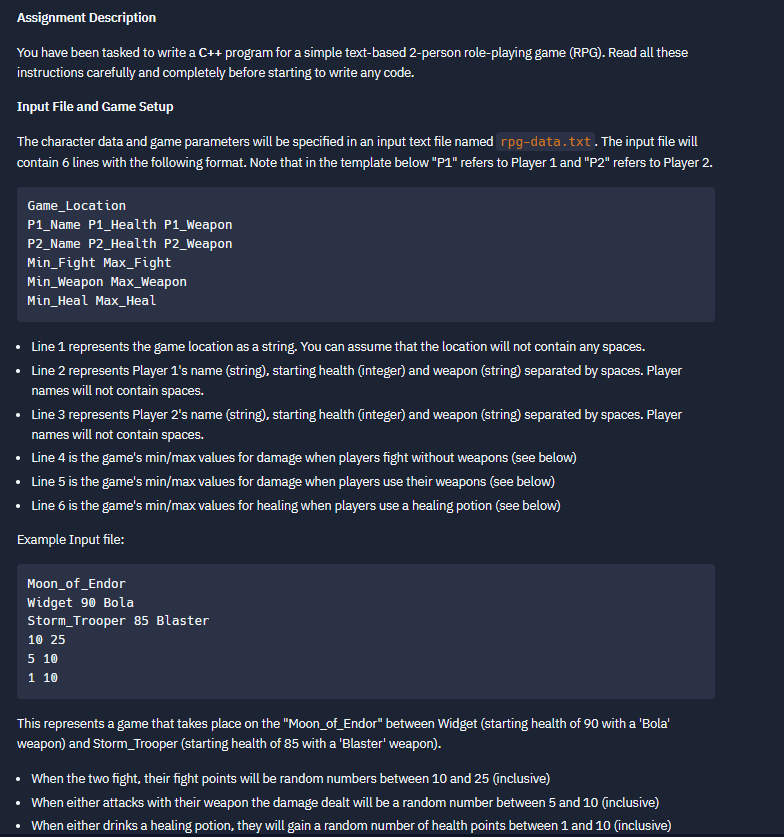
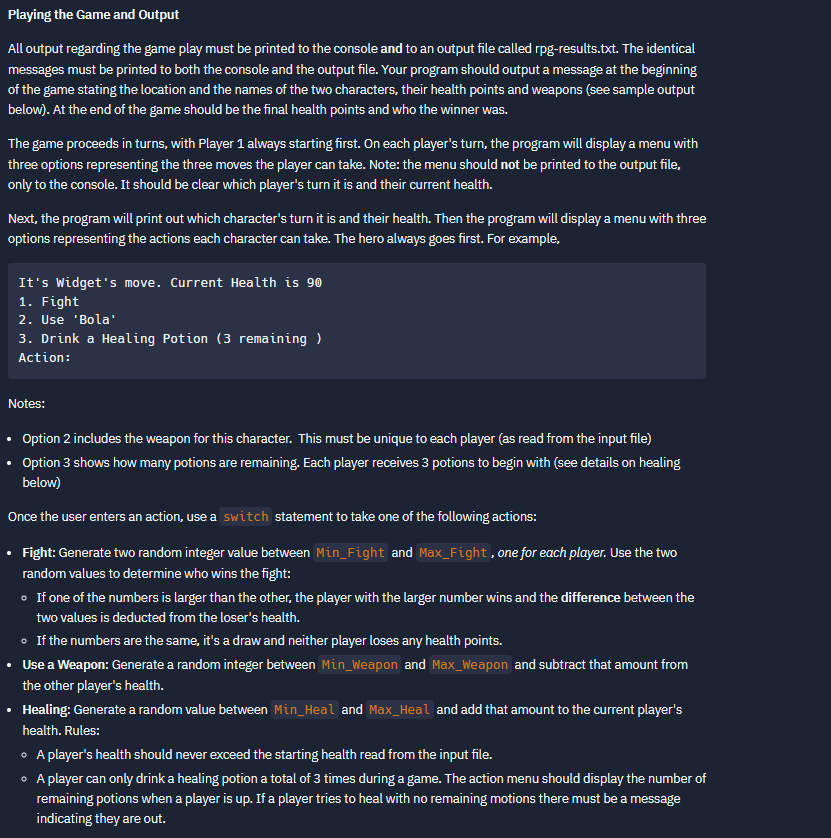
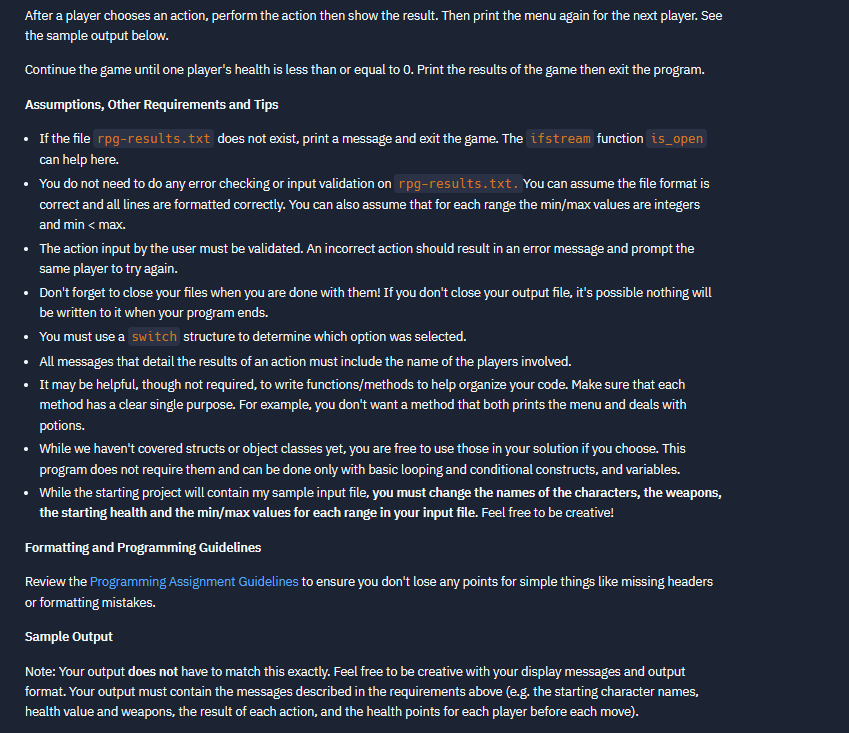
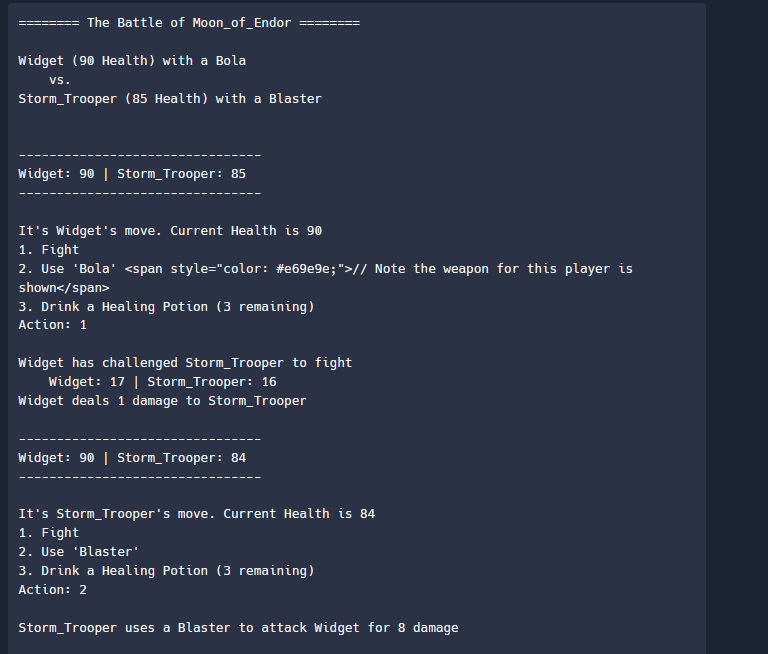
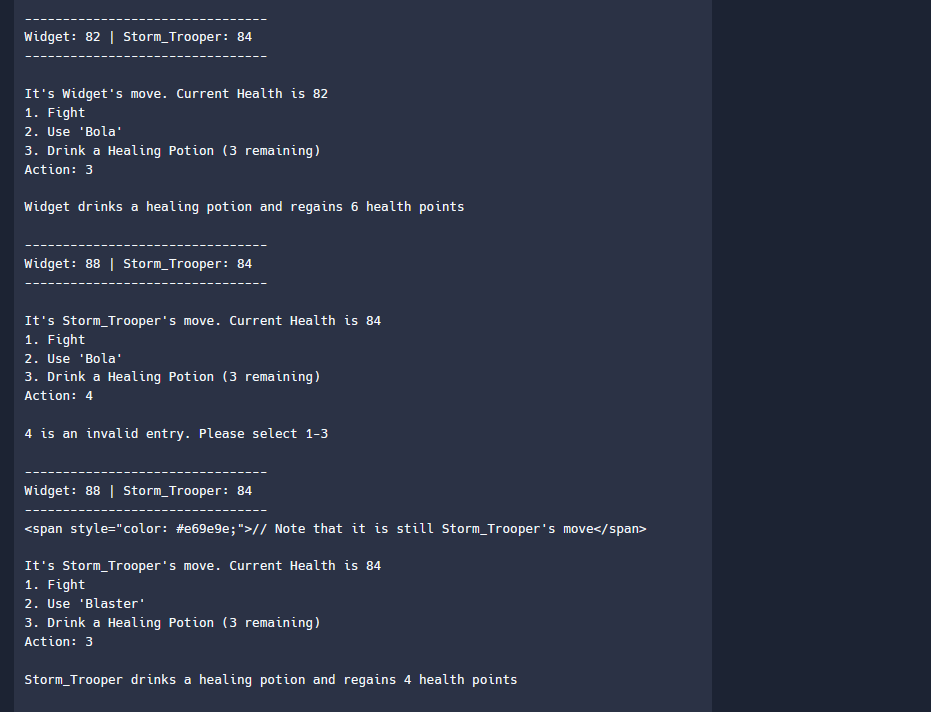
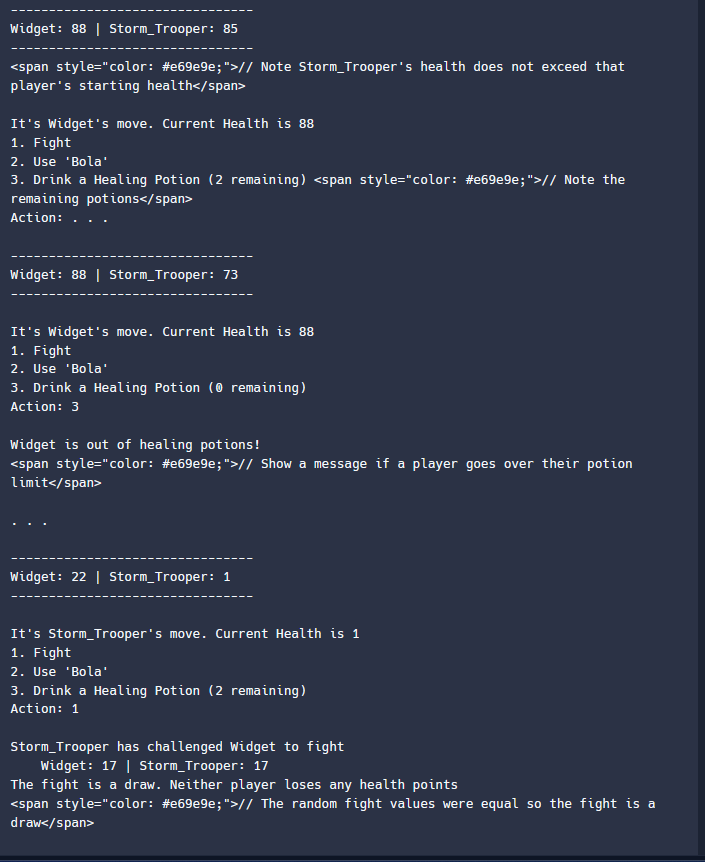
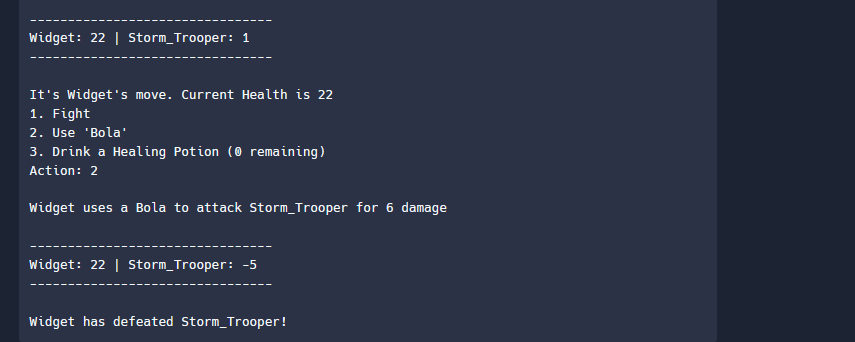
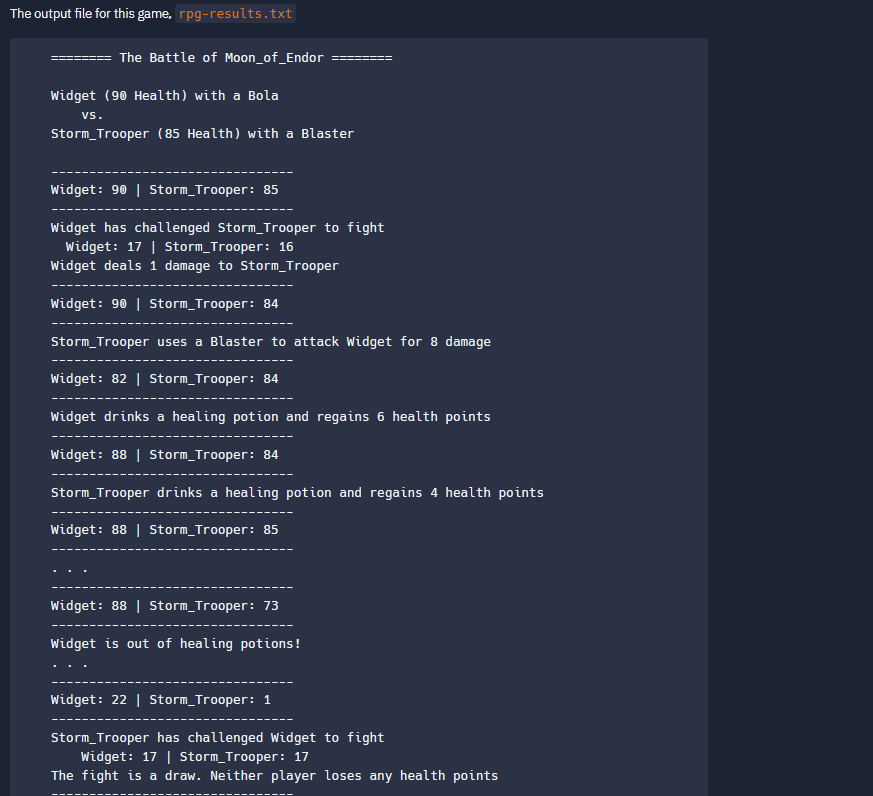
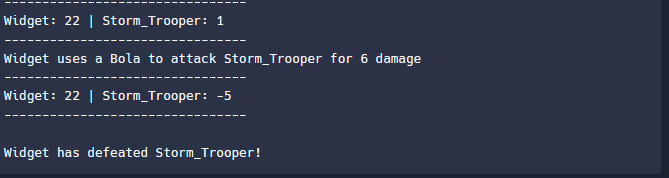
You have been tasked to write a C++ program for a simple text-based 2-person role-playing game (RPG). Read all these instructions carefully and completely before starting to write any code. Input File and Game Setup The character data and game parameters will be specified in an input text file named rpg-data. txt. The input file will contain 6 lines with the following format. Note that in the template below "P1" refers to Player 1 and "P2" refers to Player 2. Game_Location P1_Name P1_Health P1_Weapon P2_Name P2_Health P2_Weapon Min_Fight Max_Fight Min_Weapon Max_Weapon Min_Heal Max_Heal - Line 1 represents the game location as a string. You can assume that the location will not contain any spaces. - Line 2 represents Player 1's name (string), starting health (integer) and weapon (string) separated by spaces. Player names will not contain spaces. - Line 3 represents Player 2's name (string), starting health (integer) and weapon (string) separated by spaces. Player names will not contain spaces. - Line 4 is the game's min/max values for damage when players fight without weapons (see below) - Line 5 is the game's min/max values for damage when players use their weapons (see below) - Line 6 is the game's min/max values for healing when players use a healing potion (see below) Example Input file: Moon_of_Endor Widget 90 Bola Storm_Trooper 85 Blaster 1025 510 110 This represents a game that takes place on the "Moon_of_Endor" between Widget (starting health of 90 with a 'Bola' weapon) and Storm_Trooper (starting health of 85 with a 'Blaster' weapon). - When the two fight, their fight points will be random numbers between 10 and 25 (inclusive) - When either attacks with their weapon the damage dealt will be a random number between 5 and 10 (inclusive) - When either drinks a healing potion, they will gain a random number of health points between 1 and 10 (inclusive)
Playing the Game and Output All output regarding the game play must be printed to the console and to an output file called rpg-results.txt. The identical messages must be printed to both the console and the output file. Your program should output a message at the beginning of the game stating the location and the names of the two characters, their health points and weapons (see sample output below). At the end of the game should be the final health points and who the winner was. The game proceeds in turns, with Player 1 always starting first. On each player's turn, the program will display a menu with three options representing the three moves the player can take. Note: the menu should not be printed to the output file, only to the console. It should be clear which player's turn it is and their current health. Next, the program will print out which character's turn it is and their health. Then the program will display a menu with three options representing the actions each character can take. The hero always goes first. For example, It's Widget's move. Current Health is 90 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion ( 3 remaining ) Action: Notes: - Option 2 includes the weapon for this character. This must be unique to each player (as read from the input file) - Option 3 shows how many potions are remaining. Each player receives 3 potions to begin with (see details on healing below) Once the user enters an action, use a switch statement to take one of the following actions: - Fight: Generate two random integer value between Min_Fight and Max_Fight, one for each player. Use the two random values to determine who wins the fight: - If one of the numbers is larger than the other, the player with the larger number wins and the difference between the two values is deducted from the loser's health. - If the numbers are the same, it's a draw and neither player loses any health points. - Use a Weapon: Generate a random integer between Min_Weapon and Max_Weapon and subtract that amount from the other player's health. - Healing: Generate a random value between Min_Heal and Max_Heal and add that amount to the current player's health. Rules: - A player's health should never exceed the starting health read from the input file. - A player can only drink a healing potion a total of 3 times during a game. The action menu should display the number of remaining potions when a player is up. If a player tries to heal with no remaining motions there must be a message indicating they are out.
After a player chooses an action, perform the action then show the result. Then print the menu again for the next player. See the sample output below. Continue the game until one player's health is less than or equal to 0. Print the results of the game then exit the program. Assumptions, Other Requirements and Tips - If the file rpg-results. txt does not exist, print a message and exit the game. The ifstream function can help here. - You do not need to do any error checking or input validation on rpg-results. txt. You can assume the file format is correct and all lines are formatted correctly. You can also assume that for each range the min/max values are integers and min<max - The action input by the user must be validated. An incorrect action should result in an error message and prompt the same player to try again. - Don't forget to close your files when you are done with them! If you don't close your output file, it's possible nothing will be written to it when your program ends. - You must use a switch structure to determine which option was selected. - All messages that detail the results of an action must include the name of the players involved. - It may be helpful, though not required, to write functions/methods to help organize your code. Make sure that each method has a clear single purpose. For example, you don't want a method that both prints the menu and deals with potions. - While we haven't covered structs or object classes yet, you are free to use those in your solution if you choose. This program does not require them and can be done only with basic looping and conditional constructs, and variables. - While the starting project will contain my sample input file, you must change the names of the characters, the weapons, the starting health and the min/max values for each range in your input file. Feel free to be creative! Formatting and Programming Guidelines Review the Programming Assignment Guidelines to ensure you don't lose any points for simple things like missing headers or formatting mistakes. Sample Output Note: Your output does not have to match this exactly. Feel free to be creative with your display messages and output format. Your output must contain the messages described in the requirements above (e.g. the starting character names, health value and weapons, the result of each action, and the health points for each player before each move).
==-===== The Battle of Moon_of_Endor ==?==== Widget ( 90 Health) with a Bola vs. Storm_Trooper ( 85 Health) with a Blaster Widget: 90 | Storm_Trooper: 85 ------------------- It's Widget's move. Current Health is 90 1. Fight 2. Use 'Bola' // Note the weapon for this player is shown 3. Drink a Healing Potion ( 3 remaining) Action: 1 Widget has challenged Storm_Trooper to fight Widget: 17 | Storm_Trooper: 16 Widget deals 1 damage to Storm_Trooper Storm_Trooper uses a Blaster to attack Widget for 8 damage It's Storm_Trooper's move. Current Health is 84 1. Fight 2. Use 'Blaster' 3. Drink a Healing Potion ( 3 remaining) Action: 2
Widget: 82 | Storm_Trooper: 84 It's Widget's move. Current Health is 82 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion (3 remaining) Action: 3 Widget drinks a healing potion and regains 6 health points Widget: 88 | Storm_Trooper: 84 It's Storm_Trooper's move. Current Health is 84 1. Fight 2. Use "Bola' 3. Drink a Healing Potion (3 remaining) Action: 4 4 is an invalid entry. Please select 1?3 Widget: 88 | Storm_Trooper: 84 // Note that it is still Storm_Trooper's move </ spans It's Storm_Trooper's move. Current Health is 84 1. Fight 2. Use "Blaster" 3. Drink a Healing Potion ( 3 remaining) Action: 3 Storm_Trooper drinks a healing potion and regains 4 health points
Widget: 88 | Storm_Trooper: 85 // Note Storm_Trooper's health does not exceed that player's starting health It's Widget's move. Current Health is 88 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion (2 remaining) >// Note the remaining potions </ span> Action: . . Widget: 88 | Storm_Trooper: 73 It's Widget's move. Current Health is 88 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion (0 remaining) Action: 3 Widget is out of healing potions! // Show a message if a player goes over their potion limit </ span > Widget: 22 | Storm_Trooper: 1 It's Storm_Trooper's move. Current Health is 1 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion (2 remaining) Action: 1 Storm_Trooper has challenged Widget to fight Widget: 17 | Storm_Trooper: 17 The fight is a draw. Neither player loses any health points // The random fight values were equal so the fight is a draw>
Widget: 22 | Storm_Trooper: 1 It's Widget's move. Current Health is 22 1. Fight 2. Use 'Bola' 3. Drink a Healing Potion (0 remaining) Action: 2 Widget uses a Bola to attack Storm_Trooper for 6 damage Widget: 22 | Storm_Trooper: -5 Widget has defeated storm_Trooper!
output tile for this game, rpg-results. txt
Widget: 22 | Storm_Trooper: 1 Widget uses a Bola to attack Storm_Trooper for 6 damage Widget: 22 | Storm_Trooper: -5 Widget has defeated Storm_Trooper!